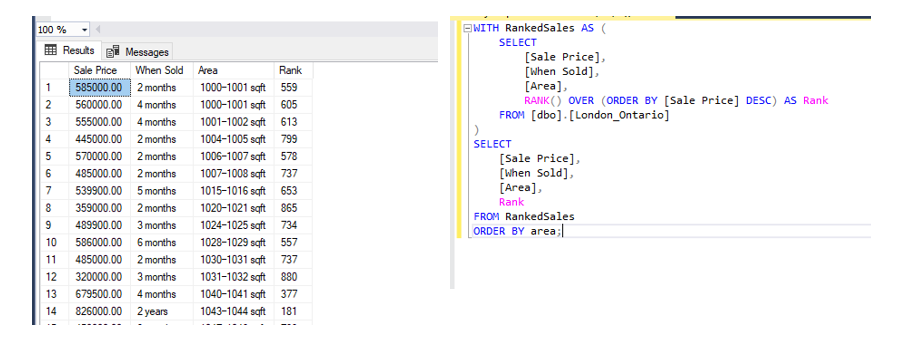
When working with relational databases, leveraging Common Table Expressions (CTEs) and ranking functions like RANK() can unlock significant potential for analyzing and organizing your data. This blog post will break down the concept of CTEs, the RANK() function, and how they can be combined effectively.
What is a Common Table Expression (CTE)?
A Common Table Expression (CTE) is a temporary result set defined within the scope of a single SELECT
, INSERT
, UPDATE
, or DELETE
statement. CTEs simplify complex queries by breaking them into manageable chunks. They’re particularly useful for improving readability and debugging complex SQL code.
Syntax:
CTE_Name AS (
-- SQL query
)
SELECT * FROM CTE_Name;
Key Features of CTEs:
- Readability: CTEs break down queries into logical parts.
- Reusability: Once defined, the CTE can be used multiple times within the main query.
- Performance: While CTEs do not inherently improve performance, their use can simplify query structure and enable optimization.
What is the RANK() Function?
The RANK() function is a window function in SQL that assigns a unique rank to each row within a partition based on a specified order. Unlike the ROW_NUMBER()
function, RANK()
can assign the same rank to rows with equal values. The next rank after a tie will skip, ensuring unique rank progression.
Syntax:
RANK() OVER (PARTITION BY [column_name] ORDER BY [column_name] ASC|DESC)
Key Features of RANK():
- Ties Handling: Rows with the same values receive the same rank.
- Order Flexibility: You can sort the rank by multiple columns.
- Custom Grouping: Combine it with
PARTITION BY
to rank within groups.
Combining CTEs and RANK(): A Practical Example
Let’s analyze the sales data of homes in London, Ontario. We’ll use a CTE to calculate the rank of each sale based on the sale price, and then query this result set to analyze trends.
Query Example:
WITH RankedSales AS (
SELECT
[Sale Price],
[When Sold],
[Area],
RANK() OVER (ORDER BY [Sale Price] DESC) AS Rank
FROM [dbo].[London_Ontario]
)
SELECT
[Sale Price],
[When Sold],
[Area],
Rank
FROM RankedSales
ORDER BY Area;
How the Query Works:
- CTE Definition (
WITH RankedSales AS
)- The CTE,
RankedSales
, calculates the rank of each sale based onSale Price
in descending order. - The
RANK()
function assigns a rank where the most expensive property receivesRank = 1
.
- The CTE,
- Main Query:
- After defining the CTE, the main query retrieves the ranked data.
- The results are then sorted by the
Area
column for analysis.
Sample Use Case: Real Estate Analysis
Scenario:
A real estate analyst wants to understand the pricing trends across different areas in London, Ontario, starting with the most expensive properties.
Benefits of This Query:
- Clarity: By calculating ranks in a separate step, the query is easier to read and modify.
- Insightful Analysis: Sorting by
Area
enables quick identification of high-value zones. - Custom Metrics: The approach can be extended with additional calculated fields or filters.
Final Thoughts
CTEs and window functions like RANK()
are indispensable tools in a SQL developer’s arsenal. They simplify complex queries while offering advanced data manipulation capabilities. In this example, combining them allows for intuitive and flexible analysis of ranked sales data.
Start incorporating these techniques into your queries to unlock the full potential of SQL for your data analysis needs. 🚀